Image plugin for ProseMirror with drop & paste handling, easy uploading, alignment selector and title
ProseMirrorImage HandlingDrag and DropFile UploadPlugin Development
By ViktorMonday, January 11, 2021
What's this about?
A ProseMirror image plugin with a lot of features:
- Optional image title
- Customizable image overlay
- Image alignment ( center, full width, left and right are default )
- Easy image uploading trough a HTTP endpoint
- Optionally removing deleted images
- Image drop & data URL paste handling
Check it out! The editor below does not upload the images anywhere, just inserts the dataURI into the ProseMirror document, which can freeze the browser up, it works fine if you host the images.
Try out with this image, drag it in or save it and pick it with the file selector.
The code for this post is here
How to use?
- Install the plugin: npm i -S prosemirror-image-plugin
- Import defaultSettings from the plugin ( and modify it if you want )
- Update the image node in the ProseMirror schema to have all the necessary properties
- Initialize the editor with the plugin
In codespeak:
1import { schema } from "prosemirror-schema-basic"; 2import { EditorState } from "prosemirror-state"; 3import { EditorView } from "prosemirror-view"; 4import { defaultSettings } from "prosemirror-image-plugin"; 5 6// Update your settings here! 7const imageSettings = {...defaultSettings}; 8 9const imageSchema = new Schema({ 10 nodes: updateImageNode(schema.spec.nodes, { 11 ...imageSettings, 12 }), 13 marks: schema.spec.marks, 14}); 15 16const initialDoc = { 17 content: [ 18 { 19 content: [ 20 { 21 text: "Start typing!", 22 type: "text", 23 }, 24 ], 25 type: "paragraph", 26 }, 27 ], 28 type: "doc", 29}; 30 31const state = EditorState.create({ 32 doc: imageSchema.nodeFromJSON(initialDoc), 33 plugins: [ 34 ...exampleSetup({ 35 schema: imageSchema, 36 menuContent: menu, 37 }), 38 imagePlugin(imageSchema, { ...imageSettings }), 39 ], 40}); 41 42const view: EditorView = new EditorView(document.getElementById("editor"), { 43 state, 44});
You might also want to add
1display: flow-root;
to the editor style in order to have correct floating images.
After that you need to add some CSS from here and voila! You have better images now, and the world just got a bit better.
You can check out the docs at https://gitlab.com/emergence-engineering/prosemirror-image-plugin
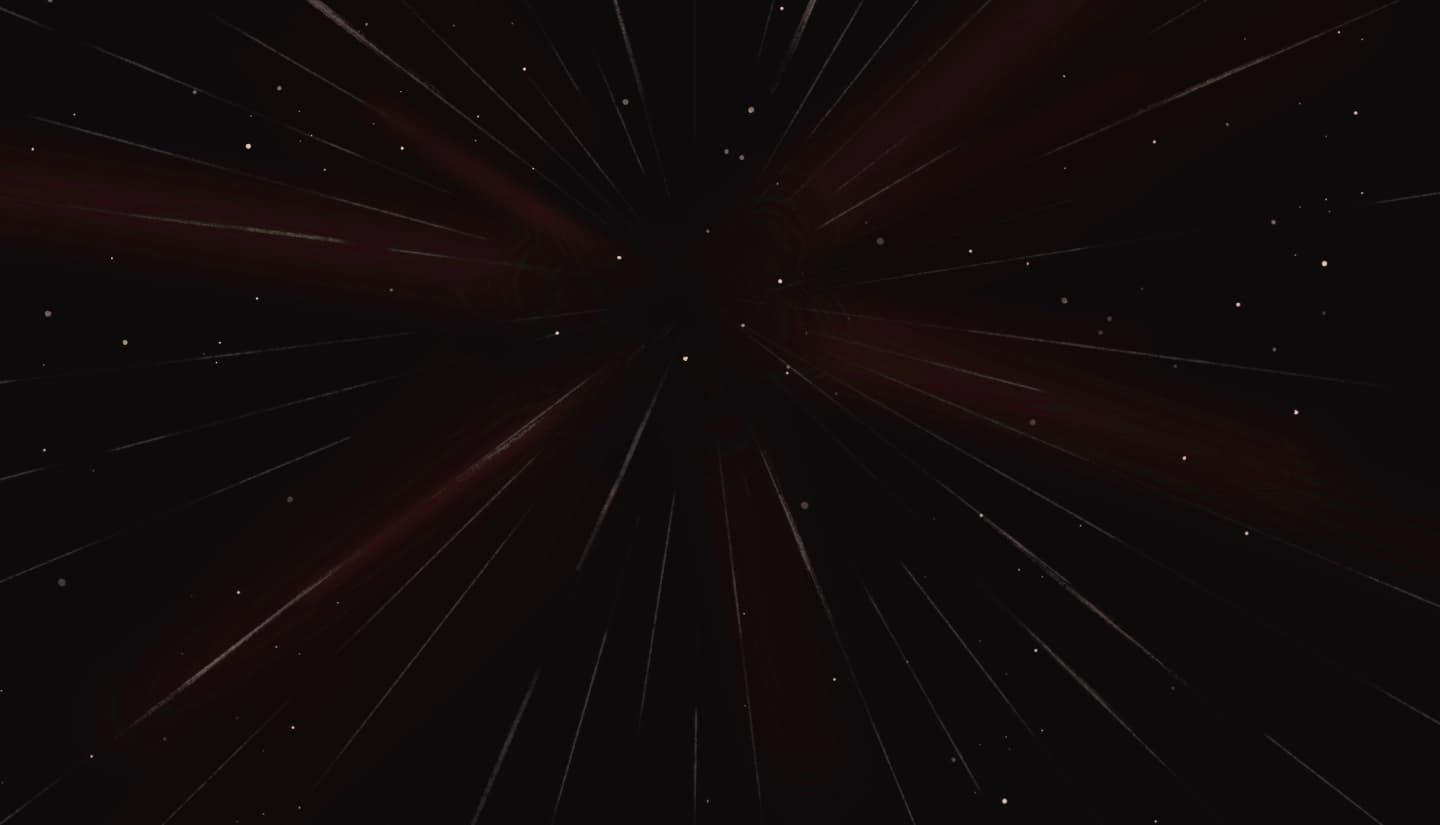
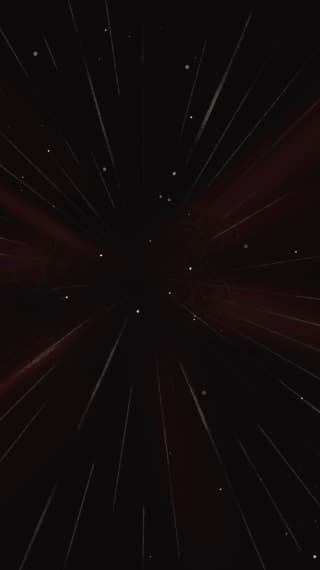
Let’s build great appstogether.
Write a message
contact@emergence-engineering.com
Schedule a call